If you are working with Qt, you may find that you need to connect one signal to multiple slots. This can be a little tricky, but fortunately, there are several ways to accomplish this.
First, let’s define what we mean by “signal” and “slot.” In Qt, a signal is an event that is emitted when some action occurs.
Exclusive Slots & Free Spins Offers:
-
500% + 150 FS 1st Deposit
-
-
For example, if you have a button in your UI and the user clicks on it, the button emits a signal indicating that it has been clicked. A slot is a function that is called when a signal is emitted. So if you have connected the button’s clicked() signal to a slot function, that function will be called whenever the button is clicked.
Now let’s look at some ways to connect one signal to multiple slots.
Method 1: Using Multiple Connections
The simplest way to connect one signal to multiple slots is to simply connect the same signal to each slot separately. For example:
“`cpp
connect(myButton, &QPushButton::clicked, myObject1, &MyClass::mySlot);
connect(myButton, &QPushButton::clicked, myObject2, &MyClass::myOtherSlot);
“`
This code connects the clicked() signal of `myButton` to two different slots: `mySlot()` in `myObject1` and `myOtherSlot()` in `myObject2`.
Method 2: Using QSignalMapper
Another way to connect one signal to multiple slots is by using a QSignalMapper object. A QSignalMapper maps an integer value (or QString) to each slot function. When the connected signal is emitted, the mapper sends the mapped value(s) as arguments to each slot.
Here’s an example:
“`cpp
QSignalMapper *mapper = new QSignalMapper(this);
connect(myButton1, &QPushButton::clicked, mapper,
static_cast(&QSignalMapper::map));
connect(myButton2, &QPushButton::clicked, mapper,
static_cast(&QSignalMapper::map));
mapper->setMapping(myButton1, 1);
mapper->setMapping(myButton2, 2);
connect(mapper, static_cast(&QSignalMapper::mapped),
myObject, &MyClass::mySlot);
connect(mapper, static_cast(&QSignalMapper::mapped),
myObject, &MyClass::myOtherSlot);
“`
In this code, we create a QSignalMapper object `mapper` and connect the clicked() signals of two buttons (`myButton1` and `myButton2`) to it. We then set up the mapper to map the integer values 1 and 2 to the buttons.
Finally, we connect the mapped() signal of the mapper to both `mySlot()` and `myOtherSlot()` in `myObject`.
Method 3: Using Qt5’s New Syntax
If you are using Qt5 or later, there is a new syntax for connecting signals to slots that makes it easy to connect one signal to multiple slots:
“`cpp
connect(myButton, &QPushButton::clicked,
[this]() { mySlot(); myOtherSlot(); });
“`
This code connects the clicked() signal of `myButton` to a lambda function that calls both `mySlot()` and `myOtherSlot()`.
Conclusion
Connecting one signal to multiple slots in Qt may seem daunting at first glance, but as we have seen there are several ways to accomplish it. Whether you use multiple connections, a QSignalMapper object or Qt5’s new syntax is up to you – choose whichever method works best for your needs.
9 Related Question Answers Found
Qt is a widely used toolkit for developing cross-platform software applications. One of the most powerful and useful features of Qt is its Signals and Slots mechanism, which allows objects to communicate with each other in a flexible and efficient manner. In this tutorial, we will explore how Signals and Slots work in Qt and how you can use them to improve the design and functionality of your applications.
Signals and slots are an important aspect of the Qt framework. They provide a way for objects to communicate with each other in a flexible and extensible manner. In this tutorial, we will explore how signals and slots work in Qt.
In Qt, signals and slots are used for communication between objects. The signal/slot mechanism is a central feature of Qt and probably the part that differs most from other toolkits. Signals and slots are very powerful and they are core to how Qt works.
If you’re looking for a way to add a little extra flair to your biscuit slots, a router is the perfect tool. Here’s how to do it:
First, mark out the biscuit slots on your workpiece. A good rule of thumb is to make the slots about half the width of the biscuit itself.
Exclusive Slots & Free Spins Offers:
500% + 150 FS 1st Deposit
Ducky Luck Review
Platinum Reels Casino Review
Diamond Reels Casino Review
Next, set up your router with a flush-trim bit.
If you are a software developer looking to create graphical user interfaces (GUIs) for your applications, then you may have come across the Qt development framework. Qt is an open-source cross-platform framework that allows developers to create powerful and intuitive GUIs for desktop, mobile, and embedded devices. One of the key features of Qt is its signal and slot mechanism.
In Qt, signals and slots are used for communication between objects. The signal/slot mechanism is a central feature of Qt and probably the part that differs most from the features provided by other frameworks. Signals and slots are very powerful and enable the objects to communicate with each other in a loosely coupled way.
Signals and slots is a Qt feature that lets you automatically connect UI elements to certain signals emitted by other widgets. This connection can be made between any two objects with compatible interfaces, regardless of whether or not they inherit from QObject. When a signal is emitted, the slot functions connected to it are executed.
When it comes to developing software applications, Qt is one of the most popular frameworks out there. This open-source framework offers a wide range of features that make it easy for developers to create applications for a variety of platforms, including desktop, mobile, and embedded systems. One of the key features of Qt is its support for signals and slots, which allow developers to create powerful event-driven applications.
Slots are functions that are executed in response to signals. Signals are emitted by objects when their state changes in a way that may be interesting to other objects. Qt’s signals and slots mechanism ensures that if you connect a signal to a slot, the slot will be called with the signal’s parameters at the right time.
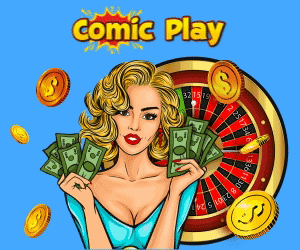