Python is an unambiguous, easy-to-read, general-purpose high-level programming language which considers paradigms of structured, procedural, and object-oriented programming.
Blackjack, also known as twenty-one, is a comparing card game between usually several players and a dealer, where each player in turn competes against the dealer, but players do not play against each other. It is played with one or more decks of 52 cards, and is the most widely played casino banking game in the world.
Exclusive BlackJack Casino Offers:
The objective of the game is to beat the dealer in one of the following ways:
Get 21 points on the player’s first two cards (called a “blackjack” or “natural”), without a dealer blackjack; Reach a final score higher than the dealer without exceeding 21; or Let the dealer draw additional cards until their hand exceeds 21 (“busted”).
Cards 2 through 10 are worth face value. Jacks, queens and kings are each worth 10. Aces can be worth 1 or 11.
The value of a hand is the sum of the point values of the individual cards. Except, a “blackjack” is the highest hand, consisting of an ace and any 10-point card, and it outranks all other 21-point hands.
Before Python 2.6 or 3.0 came out, this module was called simply “thread”.
It has been renamed in Python 3 to more accurately reflect what it does: signifying that it deals with concurrent execution of code. The “threading” module builds on the low-level features of thread to make working with threads even easier and more portable.
In order to play blackjack in Python, you must first import the “threading” module. This module will allow you to create and manage threads.
PRO TIP:If you’re looking to learn how to play Blackjack using Python, you’ll want to familiarize yourself with the rules of the game first. Then, take a look at some code samples online to get an idea of the basic structure and syntax of the game. Once you have an understanding of how the game works, you can create your own code that will let you play Blackjack in Python.
Next, you will need to create a Deck class and a Card class. These classes will be used to represent a deck of cards and an individual card, respectively.
The Deck class will need to have two methods: shuffle() and deal(). The shuffle() method will shuffle the deck of cards using the random module.
The deal() method will deal a single card from the deck to a player.
The Card class will need two attributes: suit and rank. These attributes will represent the suit and rank of an individual card.
In addition, the Card class will need two methods: getSuit() and getRank(). These methods will return the suit and rank of an individual card, respectively.
Finally, you will need to create a Blackjack class. This class will represent a game of blackjack between one or more players and a dealer.
In addition to keeping track of players’ hands, this class will also need to have two methods: hit() and stand(). The hit() method will add another card from the deck to a player’s hand while stand() signifies that a player is finished taking cards for that particular turn.
Once you have created these classes, you are ready to play blackjack in Python! To do so, simply instantiate a Blackjack object with however many players you want (including yourself) and then call its hit() or stand() methods as appropriate during gameplay.”.
8 Related Question Answers Found
Blackjack is a popular card game that is played in casinos all over the world. The object of the game is to get as close to 21 without going over, and to beat the dealer’s hand. Python is a popular programming language that is known for its ease of use and readability.
Python is an unambiguous, easy-to-read, general-purpose high-level programming language which considers paradigms of structured, procedural, and object-oriented programming. Designing a Blackjack game in Python can be done using various methods and approaches. However, the most important thing is to come up with a basic design or blueprint of the game, which will help you in understanding the game mechanics and its flow.
Exclusive BlackJack Casino Offers:
Miami Club Casino
Miami Club Casino Review
Highway Casino
Highway Casino Review
Comic Play Casino
Comic Play Casino Review
Once you have a basic understanding of the game, you can start coding it in Python.
Python is an unambiguous, easy-to-read, general-purpose high-level programming language which considers paradigms of structured, procedural, and object-oriented programming. Python is created by Guido van Rossum and first released in 1991. It takes the form of a series of nested dictionaries with keys that are strings.
Exclusive BlackJack Casino Offers:
Miami Club Casino
Miami Club Casino Review
Highway Casino
Highway Casino Review
Comic Play Casino
Comic Play Casino Review
The most important data structures in Python are lists, tuples, and dictionaries. .
Blackjack is a popular card game that has been played for centuries. The game involves a player trying to beat the dealer by getting a hand that is worth more than the dealer’s hand without going over 21. It’s a simple yet exciting game that has made its way into the digital world.
Have you ever wanted to create your own game in Python 3? Look no further than creating a Blackjack game! In this tutorial, we will guide you through the steps of creating a simple Blackjack game using Python 3.
Blackjack is a card game in which players try to score as close to 21 without going over. It is typically played with multiple decks of cards. The player or players are dealt a hand of cards, and then take turns trying to score the highest total without going over 21.
If you are a fan of blackjack and live in or near Black Hawk, Colorado, you might be wondering if it’s possible to play the game there. The answer is a resounding yes! In fact, Black Hawk is home to several casinos that offer blackjack games for players of all skill levels.
Blackjack, also known as 21, is a popular casino game that requires skill and strategy. In this tutorial, we will explain how to play real blackjack and give you some tips on how to win. Basic Rules of Blackjack:
Exclusive BlackJack Casino Offers:
Miami Club Casino
Miami Club Casino Review
Highway Casino
Highway Casino Review
Comic Play Casino
Comic Play Casino Review
The objective of the game is to have a hand with a higher total value than the dealer’s without going over 21.
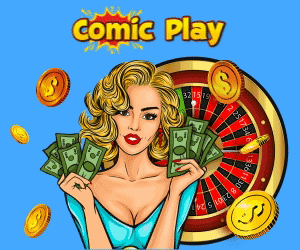