Signals and Slots are essential components in PyQt programming. They provide a simple and efficient way to manage communication between different parts of your application without having to set up complicated connections manually.
In this article, we will explore what signals and slots are in Python and how they can be used to improve the functionality of your application.
Exclusive Slots & Free Spins Offers:
-
500% + 150 FS 1st Deposit
-
-
What Are Signals?
Signals are used to indicate that an event has occurred within your application. They are emitted by objects when particular actions or state changes occur. In PyQt, signals are implemented using the QtCore.QObject class.
For example, let’s say you have a button in your application that needs to perform some action when it is clicked. You can create a signal that is emitted when the button is clicked, allowing other parts of your application to receive and respond to this event.
What Are Slots?
Slots are functions that can be connected to signals. When a signal is emitted, all connected slots will be called with any necessary arguments passed along.
Slots can be used for a wide range of purposes, such as updating UI elements or processing data from an external source. In PyQt, slots are implemented as regular Python methods.
For example, let’s say you have created a signal for a button click event. You can then create a slot function that performs some action when the button is clicked. This slot function can then be connected to the button’s signal using the connect() method.
Connecting Signals and Slots
To connect a signal to a slot in PyQt, you simply use the connect() method provided by the QObject class.
For example:
“`
button.clicked.connect(slot_function)
“`
This code connects the clicked() signal of a button object to the slot_function() method so that it will be called whenever the user clicks on the button.
The Advantages of Using Signals and Slots
Signals and slots provide several advantages over other methods of managing communication between different parts of your application.
Firstly, they make it easy to manage complex connections between different objects. Rather than having to manually set up connections between different objects, you can simply create signals and slots and let PyQt handle the rest.
Secondly, signals and slots are very efficient. They use a queued connection mechanism that ensures that the correct thread is used for each signal and slot call. This means that your application will be much faster and more responsive than if you were using other methods to manage communication.
Finally, signals and slots are very flexible. They can be used to manage a wide range of different events within your application, from user input to external data sources.
Conclusion
In conclusion, signals and slots are an essential component of PyQt programming.
By understanding how signals and slots work in Python, you can improve the functionality of your applications and create more responsive user interfaces.
10 Related Question Answers Found
Slots are a feature of Python that allows objects to be stored in a container such as a list or dict. They are similar to attributes in that they can be used to store data, but they differ in how they are accessed. Slots are accessed using the special __slots__ attribute, which is a tuple of strings.
Python is an object-oriented programming language. That means everything in Python is an object, including numbers, strings, lists, and even functions. Classes are like templates for creating objects.
Reel slots are the original slot machines that were invented over a hundred years ago. These slots are still very popular today and can be found in casinos all over the world. Reel slots are easy to play and can be a lot of fun.
Exclusive Slots & Free Spins Offers:
500% + 150 FS 1st Deposit
Ducky Luck Review
Platinum Reels Casino Review
Diamond Reels Casino Review
There are three reels in a reel slot machine.
Slots are one of the most popular casino games. They are easy to play and can be very lucrative. But what are symbols in slots?
Interactive slots are a new type of slot machines that are becoming increasingly popular in casinos. These machines are similar to traditional slots, but they offer a more interactive and engaging experience for players. Traditional slot machines are based on chance, and players have no control over the outcome of their spins.
Slots are one of the most popular casino games out there, offering players a chance to win big with every spin. But have you ever wondered what those lines mean on the reels? In this article, we’ll explore everything you need to know about slot machine paylines and how they work.
A motor slot is a type of electrical connector that is used to connect an electric motor to a power source. The most common type of motor slot is the three-phase AC motor slot, which is used to connect an AC electric motor to a three-phase power source. Other types of motor slots include the single-phase AC motor slot and the direct current (DC) motor slot.
Exclusive Slots & Free Spins Offers:
500% + 150 FS 1st Deposit
Ducky Luck Review
Platinum Reels Casino Review
Diamond Reels Casino Review
The three-phase AC motor slot is the most common type of motor slot because it can be used with any type of three-phase AC electric motor.
Computer slots are the small, rectangular openings on the front of a computer case that house various removable electronic components. These include optical drives, expansion cards, and hard drives. The number and type of slots vary depending on the form factor and model of the computer.
Exclusive Slots & Free Spins Offers:
500% + 150 FS 1st Deposit
Ducky Luck Review
Platinum Reels Casino Review
Diamond Reels Casino Review
Today, most personal computers use some form of slot to connect external devices.
Slots are the most popular games in casinos. People love to play the slots because they can win big jackpots. The slots are also easy to play and don’t require any special skills.
Exclusive Slots & Free Spins Offers:
500% + 150 FS 1st Deposit
Ducky Luck Review
Platinum Reels Casino Review
Diamond Reels Casino Review
There are two types of slots: progressive and non-progressive.
A mail slot is a thin, rectangular opening found in the door of a house or business. Mail slots are used to deliver mail directly into the building, without the need for a mailbox. The first mail slots were introduced in the early 1800s.
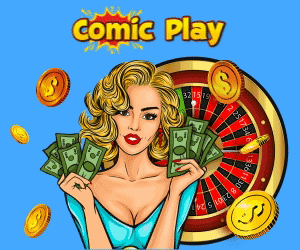