Slots in PyQt are an essential part of event-driven programming. In PyQt, a slot is a function that can be triggered by the occurrence of an event. The event can be anything from a button click to a keypress, and the slot will execute specific code based on the event that triggered it.
The concept of slots and signals is central to PyQt programming. Signals are events that are emitted by objects when a specific action occurs.
Exclusive Slots & Free Spins Offers:
-
500% + 150 FS 1st Deposit
-
-
For example, when a button is clicked, it emits a ‘clicked’ signal. Slots are functions that are called when a signal is emitted. In other words, slots receive signals and react to them accordingly.
Creating slots in PyQt is relatively straightforward. You need to define a function with the same name as the slot you want to create and decorate it with the ‘@pyqtSlot()’ decorator. The decorator tells PyQt that this function is a slot, and it should be connected to the specified signal.
For example, let’s say we have a QPushButton object named ‘button.’ We want to create a slot that will be called when the button is clicked:
“`
from PyQt5.QtCore import pyqtSlot
from PyQt5.QtWidgets import QPushButton
class MyWidget(QWidget):
def __init__(self):
super().__init__()
self.button = QPushButton(‘Click me!’)
self.button.clicked.connect(self.on_button_click)
@pyqtSlot()
def on_button_click(self):
print(‘Button clicked!’)
“`
In this example, we define a class ‘MyWidget’ that inherits from QWidget. We create an instance of QPushButton named ‘button,’ set its text to ‘Click me!’, and connect its ‘clicked’ signal to our custom slot called ‘on_button_click.’
The ‘@pyqtSlot()’ decorator tells PyQt that this function should be treated as a slot. When the button is clicked, it emits its ‘clicked’ signal, which triggers our custom slot.
The ‘on_button_click’ function then executes its code, which in this case prints ‘Button clicked!’ to the console.
Slots can also accept parameters, just like regular Python functions. To pass parameters to a slot, you need to specify them in the decorator:
“`
@pyqtSlot(int)
def on_slider_value_changed(self, value):
print(f’Slider value changed to {value}’)
“`
In this example, we define a slot called ‘on_slider_value_changed’ that accepts an integer parameter named ‘value.’ When the associated QSlider object changes its value, it emits a ‘valueChanged’ signal with the new value as an argument. The slot receives this argument and prints it to the console.
In conclusion, slots are an essential part of PyQt programming. They provide a way to handle events and execute specific code based on those events.
Creating slots is relatively straightforward in PyQt; you need to define a function with the same name as the slot and decorate it with the ‘@pyqtSlot()’ decorator. Slots can also accept parameters, making them even more versatile. By mastering slots and signals, you can create powerful and interactive GUI applications using PyQt.
10 Related Question Answers Found
Slots play an important role in Qt – a popular cross-platform application development framework. They are used for inter-object communication, which is essential for creating dynamic and responsive user interfaces. So, what exactly are slots in Qt?
If you are a software developer looking to create graphical user interfaces (GUIs) for your applications, then you may have come across the Qt development framework. Qt is an open-source cross-platform framework that allows developers to create powerful and intuitive GUIs for desktop, mobile, and embedded devices. One of the key features of Qt is its signal and slot mechanism.
Slots are functions that are executed in response to signals. Signals are emitted by objects when their state changes in a way that may be interesting to other objects. Qt’s signals and slots mechanism ensures that if you connect a signal to a slot, the slot will be called with the signal’s parameters at the right time.
Qt is a powerful development framework that allows for the creation of cross-platform applications. One of the key features of Qt is its signal and slot system, which enables communication between different objects in a program. In Qt, signals are used to emit events or notifications, while slots are used to receive and handle these events.
In Qt, signals and slots are used for communication between objects. The signal/slot mechanism is a central feature of Qt and probably the part that differs most from the features provided by other frameworks. Signals and slots are very powerful and enable the objects to communicate with each other in a loosely coupled way.
If you’re a fan of anime or manga, you may have heard of a popular series called “Hunter x Hunter,” abbreviated as “HXH.” One of the most intriguing aspects of this series is a unique ability possessed by one of the main characters, Hisoka Morow. This ability is known as “Crazy Slots.”
HXH Crazy Slots is a Nen ability that allows Hisoka to conjure a small slot machine that he can use to randomly generate various effects. Each time he uses this ability, he pulls the lever on the slot machine, and it will stop on one of several different symbols.
Signals and slots is a Qt feature that lets you automatically connect UI elements to certain signals emitted by other widgets. This connection can be made between any two objects with compatible interfaces, regardless of whether or not they inherit from QObject. When a signal is emitted, the slot functions connected to it are executed.
If you’re a gamer, you’ve probably heard the term “QD slots” thrown around. But what exactly are they? In short, QD slots are quick disconnect slots that allow you to easily swap out parts of your gaming headset, such as the microphone or the ear cups.
If you are a fan of online gambling, you may have heard of Parimatch Slots. But what exactly are they and why are they so popular? In this article, we will take a closer look at Parimatch Slots and explain everything you need to know.
If you’re a fan of online casinos, you might have come across the term “HHR slots” before. HHR stands for Historical Horse Racing, a type of game that’s gaining popularity in the US. Let’s dive into what HHR slots are and how they work.
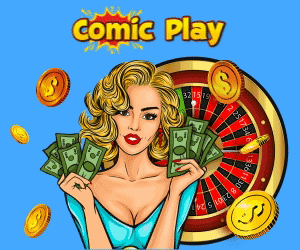